In this article, we will be creating a beautiful signup flutter form UI design, we will be using flutter textfield widgets, flutter custom check box in flutter signup ui, flutter column widgets, flutter row widgets, flutter gradient color, flutter text widgets, flutter gradient containers and much more to make the UI looks more attractive.
The widgets that we will be using in this signup flutter form UI design are all discussed in detail in the previous articles, so if you want, then you can check them.
Implementation of Flutter Signup UI Design
Let’s start implementing our design step by step, let’s start with background color.
Background Color
SafeArea( child: Scaffold( body: Container( height: double.infinity, width: double.infinity, decoration: BoxDecoration( gradient: LinearGradient( colors: [Colors.orange.shade200, Colors.orange.shade900])), ), ), )
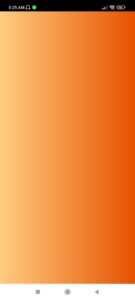
Title Text
Row( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( 'Sign', style: TextStyle( fontSize: 30, fontWeight: FontWeight.bold, color: Colors.white, shadows: [ Shadow( color: Colors.black45, offset: Offset(1, 1), blurRadius: 5) ]), ), Text( ' Me', style: TextStyle( fontSize: 30, fontWeight: FontWeight.bold, color: Colors.orange.shade700, shadows: [ Shadow( color: Colors.black45, offset: Offset(1, 1), blurRadius: 5) ]), ), Text( ' Up', style: TextStyle( fontSize: 30, fontWeight: FontWeight.bold, color: Colors.white, shadows: [ Shadow( color: Colors.black45, offset: Offset(1, 1), blurRadius: 5) ]), ) ], )
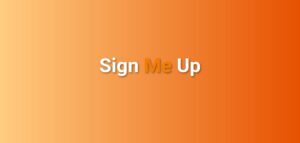
Username And Email Textfields
Let’s now add flutter text fields, we will use username, email, password, and confirm password textfield. Let’s see the code for implementing it:
TextField( style: TextStyle(color: Colors.white, fontSize: 14.5), decoration: InputDecoration( prefixIconConstraints: BoxConstraints(minWidth: 45), prefixIcon: Icon( Icons.person, color: Colors.white70, size: 22, ), border: InputBorder.none, hintText: 'Enter Username', hintStyle: TextStyle(color: Colors.white60, fontSize: 14.5), enabledBorder: OutlineInputBorder( borderRadius: BorderRadius.circular(100), borderSide: BorderSide(color: Colors.white38)), focusedBorder: OutlineInputBorder( borderRadius: BorderRadius.circular(100), borderSide: BorderSide(color: Colors.white70))), )
We have also used the enabled border and focused border constructor to decorate the borders of the text fields. We have made the border rounded and of white color. We also have used a prefix icon which is the icon that is shown before the hint text or the text that we will add after clicking on the textfield.
The email textfield is also customized using the same properties, so we only have used the username textfield code above. Let’s see both the text fields now:
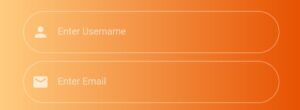
Password And Confirm Password Textfields
Password and confirm password text fields are also customized using the same properties which were used for username and email fields.
In addition, we have used the suffix property, by using it, we have specified a visibility icon that we can see at the end of both passwords and confirm password text fields. It is used to show whether the user wants to see the password while typing it or not. By clicking it, the password text will be visible, clicking it again will hide it again.
Of course, we have to implement a simple logic for this, which we have already implemented in our code. The icon will also change depending on whether the password is shown or not. Let’s see our password and confirm the password text fields:
Flutter Custom Check Box
We have created our own custom options to let the user select whether to select a male or a female option. Let’s see the code for its implementation:
Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ GestureDetector( onTap: () { setState(() { maleSelected = true; femaleSelected = false; }); }, child: Row( children: [ Container( height: 20, width: 20, alignment: Alignment.center, margin: EdgeInsets.only(right: 10), decoration: BoxDecoration( shape: BoxShape.circle, border: Border.all(color: Colors.white60)), child: maleSelected ? Container( margin: EdgeInsets.all(4), decoration: BoxDecoration( shape: BoxShape.circle, color: Colors.white70), ) : SizedBox()), Text('Male', style: TextStyle( color: Colors.white70, fontSize: 14.5)) ], ), ), GestureDetector( onTap: () { setState(() { femaleSelected = true; maleSelected = false; }); }, child: Row( children: [ Container( height: 20, width: 20, alignment: Alignment.center, margin: EdgeInsets.only(right: 10), decoration: BoxDecoration( shape: BoxShape.circle, border: Border.all(color: Colors.white60)), child: femaleSelected ? Container( margin: EdgeInsets.all(4), decoration: BoxDecoration( shape: BoxShape.circle, color: Colors.white70), ) : SizedBox()), Text('Female', style: TextStyle( color: Colors.white70, fontSize: 14.5)) ], ), ) ], )
We can see that we have used Boolean values for this, we have implemented some logic, so that whenever one option is selected, the other gets unselected automatically.
Flutter Gradient Button Container
We have used a container and customized it, we have given it a gradient background color, a child text widget, a shadow, and a circular border-radius, we also have wrapped it inside a flutter gesture detector widget so its clickable now and we can perform actions by clicking it. Let’s check the code out:
GestureDetector( onTap: () {}, child: Container( height: 53, width: double.infinity, margin: EdgeInsets.symmetric(horizontal: 30), alignment: Alignment.center, decoration: BoxDecoration( boxShadow: [ BoxShadow( blurRadius: 4, color: Colors.black12.withOpacity(.2), offset: Offset(2, 2)) ], borderRadius: BorderRadius.circular(100), gradient: LinearGradient(colors: [ Colors.orange.shade200, Colors.orange.shade900 ])), child: Text('Signup', style: TextStyle( color: Colors.white.withOpacity(.8), fontSize: 15, fontWeight: FontWeight.bold)), ), )
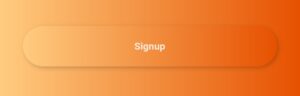
Flutter Login Container Button
Conclusion
Hope you now have a complete understanding of how to implement this eye-catching Flutter Signup UI Design. We’ve written detailed articles on the widgets that are used to create this beautiful design, so do check them out as well.
We’d also be glad to see you visit our other tutorials on Flutter app development and Python programming. Thank you for reading this one.