In this Flutter post, we will be going through how to properly use Flutter snackbar in Flutter apps. We will be implementing Flutter snackbar step by step and with a proper Flutter example to give you a better practical idea of how to use it. After reading this post, you will quickly be able to incorporate Flutter snackbar to your own Flutter applications. So let’s just dive right into its implementation.
What is Flutter Snackbar?
It is used to show some information to the user after some action takes place. The information can be like, the task is done, successfully downloaded etc. You can even give the user an option to undo the action.
Let’s now implement snackbar practically in our Flutter app.
Implementing Flutter Snackbar (Steps)
In order to use the Flutter snackbar, just follow the steps below:
Step 1: Create a Flutter Button
We will be creating a Flutter raisedbutton so after clicking it, we can see a snackbar. You can use other Flutter widgets as well like a text widget and make it clickable by wrapping it with ink well or gesture detector class. We will define the implementation of Flutter snackbar in our Flutter raisedbutton onPressed function. But first let’s just create a simple raisedbutton. See below code:
RaisedButton( onPressed: () {}, color: Colors.blue, child: Text( 'Click here to see a Snackbar', style: TextStyle(color: Colors.white), ), )
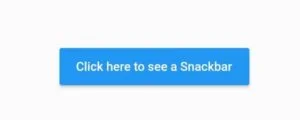
Step 2: Using Flutter Snackbar
We have to use the onPressed constructor of the raised button. It takes a function, so we will pass our Flutter snackbar in that function’s body. See below code:
ScaffoldMessenger.of(context).showSnackBar( SnackBar(content: Text('This is a snackbar')) )
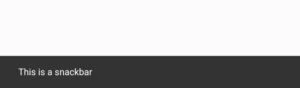
Step 3: Flutter Snackbar Customization
Let’s now customize our Flutter snackbar widget using its constructors.
Content Constructor
As you can see in the above example code, we have used the content constructor of snackbar class. It takes a widget which means we can pass image, row, column or some other widget to it.
Action Constructor
We also have an action constructor which takes snack bar action widget. See below code:
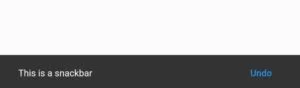
onPressed: () { ScaffoldMessenger.of(context).hideCurrentSnackBar(); }
Background Color Constructor
In order to change the background color of Flutter snackbar, we have to use the background color constructor of snackbar class. It takes color so we will pass a blue color to it for demonstration. See below code:
backgroundColor: Colors.blue
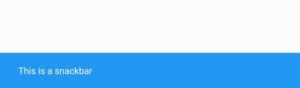
Duration Constructor
It specifies the amount of time snackbar will be visible on the screen. Duration constructor is used to customize that time. See below code:
duration: Duration(seconds: 2)
Conclusion
To conclude, hope you now have learned how to properly use and customize Flutter snackbar in Flutter apps. I would love to see how you use it. Do share your experience with us. Thank you for reading this Flutter post.