In this article, we will understand how to create a flutter Instagram news feed UI in flutter, we will customize it to make it a little different. We will be using list view builder, row widgets, column widgets, container widgets, images, icons that we have covered in our previous articles and will try to implement each of them in that news feed UI.
We will try to implement the full Instagram app UI, so we will divide it in articles. We are covering the news feed UI in this article and in the next articles, we will cover the profile UI, chats UI, login, signup UI etc. So, don’t miss them. Let’s get right into our Instagram news feed.
Instagram News Feed UI
This is the design that we will achieve by the end of this article. Let’s now understand how its implemented.
Implementation
Let’s start by implementing from top. Let’s see the implementations.
App Bar
AppBar( centerTitle: true, backgroundColor: Colors.transparent, elevation: 0, title: Text( 'Zeesta', style: TextStyle( fontSize: 22, fontWeight: FontWeight.bold, fontStyle: FontStyle.italic, color: Colors.white), ), actions: [ Icon(Icons.message_outlined, color: Colors.white, size: 18), SizedBox( width: 8, ), Icon(Icons.settings, color: Colors.white, size: 19), SizedBox( width: 15, ) ], )
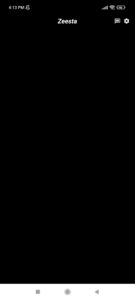
Body Of Scaffold
body:Container( height: double.infinity, width: double.infinity)
List View Builder
The list of images with icons, text etc. are build using the listview builder. Let’s see how each item is built.
Item Count
We have specified item count to 4 to get only 4 items in list as for now.
Item Builder
itemBuilder: (context, index) { return Container( margin: EdgeInsets.only(bottom: 10),)
From the item builder, we are returning a container with a bottom margin of 10. Let’s see what we have used in the child of this container.
Column
We have used a column widget inside that container.
First Child
First child of column will be the small circular image with name and the vertical three dots and so on.
Container( height: 50, child: Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ Container( width: 200, child: Row( children: [ Container( margin: EdgeInsets.symmetric(horizontal: 5), padding: EdgeInsets.all(2.5), decoration: BoxDecoration( borderRadius: BorderRadius.circular(50), color: Colors.grey.shade800), child: Container( padding: EdgeInsets.all(1), decoration: BoxDecoration( borderRadius: BorderRadius.circular(50), color: Colors.black), child: Container( height: 40, width: 40, decoration: BoxDecoration( borderRadius: BorderRadius.circular(50), image: DecorationImage( fit: BoxFit.cover, image: AssetImage( 'assets/pic${imgUrl[index + 4]}'))), ), ), ), SizedBox( height: 5, ), Text(imgNames[index], style: TextStyle( color: Colors.white, fontSize: 13, fontWeight: FontWeight.w800)) ], ), ), Container( width: 50, child: Icon(Icons.more_vert_outlined, size: 18, color: Colors.white)) ], ), )
Second Child
The second child is the waterfall image that you can see in the mentioned design.
Container( color: Colors.black, height: 210, width: MediaQuery.of(context).size.width, child: Image.asset('assets/pic${imgUrl[index + 4]}', fit: BoxFit.cover), )
Third Child
Third child is the row widget and inside it we can see two children, first one is heart, chat and send icon and the second one is the bookmark icon. All of them have a color of white.
Container( height: 30, child: Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ Container( width: 200, child: Row( children: [ SizedBox(width: 10), Icon( Icons.favorite_border_outlined, size: 22, color: Colors.white, ), SizedBox(width: 6), Icon(Icons.comment_outlined, size: 20, color: Colors.white), SizedBox(width: 6), Icon(Icons.send_outlined, size: 21, color: Colors.white) ], ), ), Container( width: 50, child: Icon(Icons.bookmark_border_outlined, size: 22, color: Colors.white)) ], ), ),
Fourth Child
In the next child of our column widget, we have a number of likes text.
Align( alignment: Alignment.centerLeft, child: Padding( padding: const EdgeInsets.only(left: 10), child: Text('198,459 likes', style: TextStyle( fontSize: 11, color: Colors.white, fontWeight: FontWeight.w600)), ))
Fifth Child
After that we have a row having both children of text.
Align( alignment: Alignment.centerLeft, child: Padding( padding: const EdgeInsets.only(left: 10), child: Row( children: [ Text(imgNames[index], style: TextStyle( fontSize: 13, color: Colors.white, fontWeight: FontWeight.w800)), SizedBox(width: 8), Text(captions[index], style: TextStyle( fontSize: 11, fontWeight: FontWeight.w400, color: Colors.white)), ], ), ))
Sixth Child
In the last child of column, we have a text of number of comments.
Align( alignment: Alignment.centerLeft, child: Padding( padding: const EdgeInsets.only(left: 10), child: Text('View all 5980 comments', style: TextStyle( fontSize: 12, fontWeight: FontWeight.w500, color: Colors.white)), ))
Congrats, we have successfully created our instagram news feed UI. In the next articles, we will cover other pages of instagram. Hope to see you there as well.
Conclusion
That’s all for this article, hope you enjoyed it and have learnt a lot from it. We would be looking forward for your response. Hope to see you in our next articles in which we will dig deep into other amazing widgets. Thank you for reading it.
You really make it seem so easy with your presentation but I find this topic to be actually
something which I think I would never understand. It seems too complex and very
broad for me. I am looking forward for your next post, I’ll try to get the hang of it!
Thanks for the support. Do visit my other posts.