In this Flutter post, we will be implementing Flutter image picker practically with the help of an easy Flutter example. Providing step by step explanation of how to use Flutter image picker and how we can be pick images and show it in our Flutter app.
After reading this post, you will be able to use Flutter image picker with ease.
What is Flutter Image Picker?
Flutter image picker is a package that is used to pick images using camera or gallery. We will see a proper example in which we will take images from both gallery and camera. We will then show it in our app to make sure the images are picked properly. So let’s just dive right implementing it in our app.
Implementing Flutter Image Picker (Easy Example)
See the below steps in which each and every detail is covered about picking and showing image.
Step 1: Import Image Picker Package
image_picker: ^0.8.5+3
First we have to import the package. You can click here to get the latest version of it. Given above is the current latest version.
Note:
If this version gives some error while building apk then give the below version a try.
image_picker: ^0.7.5+1
Now add it to your pubspec.yaml file dependencies like shown in the above image. After that, just click save so this package can be imported in your Flutter app.
Step 2: Fetch Image From Camera
To order to fetch image from camera, first we have to use a function that when triggered, it will get the image from camera. We have to declare a file variable so we can store the fetched image in it. See below code for proper implementation.
ImagePicker _imagePicker = ImagePicker(); File? imageFile; getImageFromGallery() async { var imageSource = await _imagePicker.getImage(source: ImageSource.camera); setState(() { imageFile = File(imageSource!.path); }); }
GestureDetector( onTap: () { getImageFromGallery(); }, child: Container( height: 200, width: 200, decoration: BoxDecoration(color: Colors.grey.shade300, shape: BoxShape.circle), child: imageFile == null ? Icon( Icons.camera, size: 30, ) : ClipRRect( borderRadius: BorderRadius.circular(300), child: Image.file( imageFile!, fit: BoxFit.cover, ), ), ), )
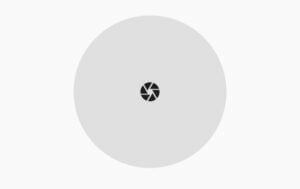
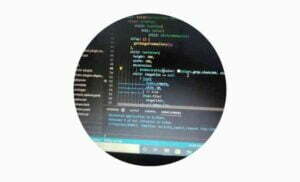
Step 3: Fetch Image From Gallery
In order to fetch image from gallery, you have to use the same above process. Just change the image source. See below code:
var imageSource = await _imagePicker.getImage(source: ImageSource.gallery);
Conclusion
To conclude, we have practically implemented Flutter image picker in our Flutter app with the help of proper and easy Flutter example. Thank you for reading this post.